1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139 140 141 142 143 144 145 146 147 148 149 150 151 152 153 154 155 156 157 158 159 160 161 162 163 164 165 166 167 168 169 170 171 172 173 174 175 176 177 178 179 180 181 182 183 184 185 186
//! OpenAPI support for Poem.
//!
//! `Poem-openapi` allows you to easily implement APIs that comply with the
//! `OpenAPIv3` specification. It uses procedural macros to generate a lots of
//! boilerplate code, so that you only need to focus on the more
//! important business implementations.
//!
//! # Table of contents
//!
//! - [Features](#features)
//! - [Quickstart](#quickstart)
//! - [Crate features](#crate-features)
//!
//! ## Features
//!
//! * **Type safety** If your codes can be compiled, then it is fully compliant
//! with the `OpenAPI v3` specification.
//! * **Rustfmt friendly** Do not create any DSL that does not conform to Rust's
//! syntax specifications.
//! * **IDE friendly** Any code generated by the procedural macro will not be
//! used directly.
//! * **Minimal overhead** All generated code is necessary, and there is almost
//! no overhead.
//!
//! ## Quickstart
//!
//! Cargo.toml
//!
//! ```toml
//! [package]
//! name = "helloworld"
//! version = "0.1.0"
//! edition = "2021"
//!
//! [dependencies]
//! poem = "1.2"
//! poem-openapi = { version = "1.2", features = ["swagger-ui"] }
//! tokio = { version = "1", features = ["macros", "rt-multi-thread"] }
//! ```
//!
//! main.rs
//!
//! ```ignore
//! use poem::{listener::TcpListener, Route, Server};
//! use poem_openapi::{payload::PlainText, OpenApi, OpenApiService};
//!
//! struct Api;
//!
//! #[OpenApi]
//! impl Api {
//! /// Hello world
//! #[oai(path = "/", method = "get")]
//! async fn index(&self) -> PlainText<&'static str> {
//! PlainText("Hello World")
//! }
//! }
//!
//! let api_service =
//! OpenApiService::new(Api, "Hello World", "1.0").server("http://localhost:3000");
//! let ui = api_service.swagger_ui();
//! let app = Route::new().nest("/", api_service).nest("/docs", ui);
//!
//! # tokio::runtime::Runtime::new().unwrap().block_on(async {
//! Server::new(TcpListener::bind("127.0.0.1:3000"))
//! .run(app)
//! .await;
//! # });
//! ```
//!
//! ## Check it
//!
//! Open your browser at [http://127.0.0.1:3000](http://127.0.0.1:3000).
//!
//! You will see the plaintext response as:
//!
//! ```text
//! Hello World
//! ```
//!
//! ## Interactive API docs
//!
//! Now go to [http://127.0.0.1:3000/docs](http://127.0.0.1:3000/docs).
//!
//! You will see the automatic interactive API documentation (provided by
//! [Swagger UI](https://github.com/swagger-api/swagger-ui)):
//!
//! 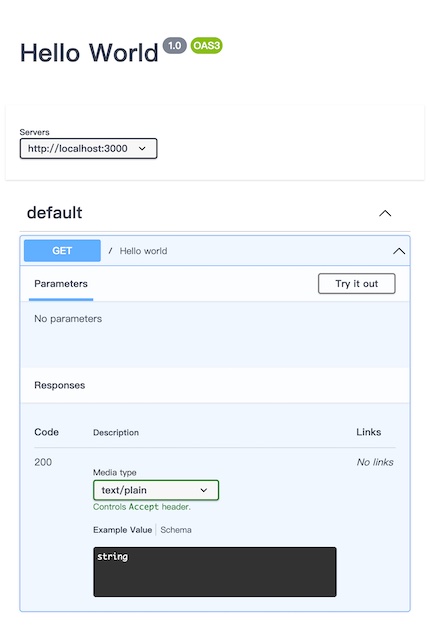
//!
//! ## Crate features
//!
//! To avoid compiling unused dependencies, Poem gates certain features, some of
//! which are disabled by default:
//!
//! | Feature | Description |
//! |------------|-----------------------------------------------------------------------|
//! | chrono | Integrate with the [`chrono` crate](https://crates.io/crates/chrono) |
//! | time | Integrate with the [`time` crate](https://crates.io/crates/time). |
//! | humantime | Integrate with the [`humantime` crate](https://crates.io/crates/humantime) |
//! | swagger-ui | Add swagger UI support |
//! | rapidoc | Add RapiDoc UI support |
//! | redoc | Add Redoc UI support |
//! | email | Support for email address string |
//! | hostname | Support for hostname string |
//! | uuid | Integrate with the [`uuid` crate](https://crates.io/crates/uuid)|
//! | url | Integrate with the [`url` crate](https://crates.io/crates/url) |
//! | bson | Integrate with the [`bson` crate](https://crates.io/crates/bson) |
//! | rust_decimal | Integrate with the [`rust_decimal` crate](https://crates.io/crates/rust_decimal) |
//! | static-files | Support for static file response |
#![doc(html_favicon_url = "https://raw.githubusercontent.com/poem-web/poem/master/favicon.ico")]
#![doc(html_logo_url = "https://raw.githubusercontent.com/poem-web/poem/master/logo.png")]
#![forbid(unsafe_code)]
#![deny(private_in_public, unreachable_pub)]
#![cfg_attr(docsrs, feature(doc_cfg))]
#![warn(rustdoc::broken_intra_doc_links)]
#![warn(missing_docs)]
/// Macros to help with building custom payload types.
#[macro_use]
pub mod macros;
pub mod auth;
pub mod error;
pub mod param;
pub mod payload;
#[doc(hidden)]
pub mod registry;
mod response;
pub mod types;
#[doc(hidden)]
pub mod validation;
mod base;
mod openapi;
#[cfg(any(
feature = "swagger-ui",
feature = "rapidoc",
feature = "redoc",
feature = "openapi-explorer"
))]
mod ui;
pub use base::{
ApiExtractor, ApiExtractorType, ApiResponse, ExtractParamOptions, OAuthScopes, OpenApi,
OperationId, ResponseContent, Tags, Webhook,
};
pub use openapi::{
ContactObject, ExternalDocumentObject, ExtraHeader, LicenseObject, OpenApiService, ServerObject,
};
#[doc = include_str!("docs/request.md")]
pub use poem_openapi_derive::ApiRequest;
#[doc = include_str!("docs/response.md")]
pub use poem_openapi_derive::ApiResponse;
#[doc = include_str!("docs/enum.md")]
pub use poem_openapi_derive::Enum;
#[doc = include_str!("docs/multipart.md")]
pub use poem_openapi_derive::Multipart;
#[doc = include_str!("docs/newtype.md")]
pub use poem_openapi_derive::NewType;
#[doc = include_str!("docs/oauth_scopes.md")]
pub use poem_openapi_derive::OAuthScopes;
#[doc = include_str!("docs/object.md")]
pub use poem_openapi_derive::Object;
#[doc = include_str!("docs/openapi.md")]
pub use poem_openapi_derive::OpenApi;
#[doc = include_str!("docs/response_content.md")]
pub use poem_openapi_derive::ResponseContent;
#[doc = include_str!("docs/security_scheme.md")]
pub use poem_openapi_derive::SecurityScheme;
#[doc = include_str!("docs/tags.md")]
pub use poem_openapi_derive::Tags;
#[doc = include_str!("docs/union.md")]
pub use poem_openapi_derive::Union;
#[doc = include_str!("docs/webhook.md")]
pub use poem_openapi_derive::Webhook;
pub use validation::Validator;
#[doc(hidden)]
pub mod __private {
pub use mime;
pub use poem;
pub use serde;
pub use serde_json;
pub use crate::base::UrlQuery;
}