poem_openapi/lib.rs
1//! OpenAPI support for Poem.
2//!
3//! `Poem-openapi` allows you to easily implement APIs that comply with the
4//! `OpenAPIv3` specification. It uses procedural macros to generate a lots of
5//! boilerplate code, so that you only need to focus on the more
6//! important business implementations.
7//!
8//! # Table of contents
9//!
10//! - [Features](#features)
11//! - [Quickstart](#quickstart)
12//! - [Crate features](#crate-features)
13//!
14//! ## Features
15//!
16//! * **Type safety** If your codes can be compiled, then it is fully compliant
17//! with the `OpenAPI v3` specification.
18//! * **Rustfmt friendly** Do not create any DSL that does not conform to Rust's
19//! syntax specifications.
20//! * **IDE friendly** Any code generated by the procedural macro will not be
21//! used directly.
22//! * **Minimal overhead** All generated code is necessary, and there is almost
23//! no overhead.
24//!
25//! ## Quickstart
26//!
27//! Cargo.toml
28//!
29//! ```toml
30//! [package]
31//! name = "helloworld"
32//! version = "0.1.0"
33//! edition = "2021"
34//!
35//! [dependencies]
36//! poem = "3"
37//! poem-openapi = { version = "5", features = ["swagger-ui"] }
38//! tokio = { version = "1", features = ["macros", "rt-multi-thread"] }
39//! ```
40//!
41//! main.rs
42//!
43//! ```ignore
44//! use poem::{listener::TcpListener, Route, Server};
45//! use poem_openapi::{payload::PlainText, OpenApi, OpenApiService};
46//!
47//! struct Api;
48//!
49//! #[OpenApi]
50//! impl Api {
51//! /// Hello world
52//! #[oai(path = "/", method = "get")]
53//! async fn index(&self) -> PlainText<&'static str> {
54//! PlainText("Hello World")
55//! }
56//! }
57//!
58//! #[tokio::main]
59//! async fn main() {
60//! let api_service =
61//! OpenApiService::new(Api, "Hello World", "1.0").server("http://localhost:3000");
62//! let ui = api_service.swagger_ui();
63//! let app = Route::new().nest("/", api_service).nest("/docs", ui);
64//!
65//! Server::new(TcpListener::bind("127.0.0.1:3000"))
66//! .run(app)
67//! .await;
68//! }
69//! ```
70//!
71//! ## Check it
72//!
73//! Open your browser at [http://127.0.0.1:3000](http://127.0.0.1:3000).
74//!
75//! You will see the plaintext response as:
76//!
77//! ```text
78//! Hello World
79//! ```
80//!
81//! ## Interactive API docs
82//!
83//! Now go to [http://127.0.0.1:3000/docs](http://127.0.0.1:3000/docs).
84//!
85//! You will see the automatic interactive API documentation (provided by
86//! [Swagger UI](https://github.com/swagger-api/swagger-ui)):
87//!
88//! 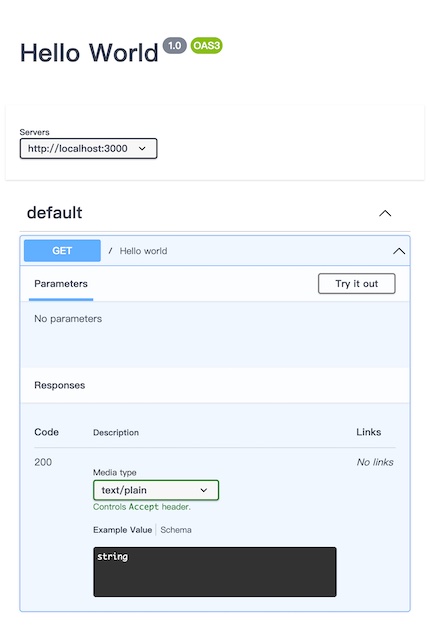
89//!
90//! ## Crate features
91//!
92//! To avoid compiling unused dependencies, Poem gates certain features, some of
93//! which are disabled by default:
94//!
95//! | Feature | Description |
96//! |--------------------|----------------------------------------------------------------------------------------|
97//! | chrono | Integrate with the [`chrono` crate](https://crates.io/crates/chrono). |
98//! | time | Integrate with the [`time` crate](https://crates.io/crates/time). |
99//! | humantime | Integrate with the [`humantime` crate](https://crates.io/crates/humantime) |
100//! | openapi-explorer | Add OpenAPI Explorer support |
101//! | swagger-ui | Add swagger UI support |
102//! | rapidoc | Add RapiDoc UI support |
103//! | redoc | Add Redoc UI support |
104//! | stoplight-elements | Add Stoplight Elements UI support |
105//! | email | Support for email address string |
106//! | hostname | Support for hostname string |
107//! | humantime | Integrate with the [`humantime` crate](https://crates.io/crates/humantime) |
108//! | uuid | Integrate with the [`uuid` crate](https://crates.io/crates/uuid) |
109//! | url | Integrate with the [`url` crate](https://crates.io/crates/url) |
110//! | geo | Integrate with the [`geo-types` crate](https://crates.io/crates/geo-types) |
111//! | bson | Integrate with the [`bson` crate](https://crates.io/crates/bson) |
112//! | rust_decimal | Integrate with the [`rust_decimal` crate](https://crates.io/crates/rust_decimal) |
113//! | prost-wkt-types | Integrate with the [`prost-wkt-types` crate](https://crates.io/crates/prost-wkt-types) |
114//! | static-files | Support for static file response |
115//! | websocket | Support for websocket |
116//! | sonic-rs | Uses [`sonic-rs`](https://github.com/cloudwego/sonic-rs) instead of `serde_json`. Pls, checkout `sonic-rs` requirements to properly enable `sonic-rs` capabilities |
117
118#![doc(html_favicon_url = "https://raw.githubusercontent.com/poem-web/poem/master/favicon.ico")]
119#![doc(html_logo_url = "https://raw.githubusercontent.com/poem-web/poem/master/logo.png")]
120#![forbid(unsafe_code)]
121#![deny(unreachable_pub)]
122#![cfg_attr(docsrs, feature(doc_cfg))]
123#![warn(rustdoc::broken_intra_doc_links)]
124#![warn(missing_docs)]
125
126/// Macros to help with building custom payload types.
127#[macro_use]
128pub mod macros;
129
130pub mod auth;
131pub mod error;
132pub mod param;
133pub mod payload;
134#[doc(hidden)]
135pub mod registry;
136mod response;
137pub mod types;
138#[doc(hidden)]
139pub mod validation;
140
141mod base;
142mod openapi;
143mod path_util;
144#[cfg(any(
145 feature = "openapi-explorer",
146 feature = "rapidoc",
147 feature = "redoc",
148 feature = "stoplight-elements",
149 feature = "swagger-ui",
150))]
151mod ui;
152
153pub use base::{
154 ApiExtractor, ApiExtractorType, ApiResponse, ExtractParamOptions, OAuthScopes, OpenApi,
155 OperationId, ParameterStyle, ResponseContent, Tags, Webhook,
156};
157pub use openapi::{
158 ContactObject, ExternalDocumentObject, ExtraHeader, LicenseObject, OpenApiService, ServerObject,
159};
160#[doc = include_str!("docs/request.md")]
161pub use poem_openapi_derive::ApiRequest;
162#[doc = include_str!("docs/response.md")]
163pub use poem_openapi_derive::ApiResponse;
164#[doc = include_str!("docs/enum.md")]
165pub use poem_openapi_derive::Enum;
166#[doc = include_str!("docs/multipart.md")]
167pub use poem_openapi_derive::Multipart;
168#[doc = include_str!("docs/newtype.md")]
169pub use poem_openapi_derive::NewType;
170#[doc = include_str!("docs/oauth_scopes.md")]
171pub use poem_openapi_derive::OAuthScopes;
172#[doc = include_str!("docs/object.md")]
173pub use poem_openapi_derive::Object;
174#[doc = include_str!("docs/openapi.md")]
175pub use poem_openapi_derive::OpenApi;
176#[doc = include_str!("docs/response_content.md")]
177pub use poem_openapi_derive::ResponseContent;
178#[doc = include_str!("docs/security_scheme.md")]
179pub use poem_openapi_derive::SecurityScheme;
180#[doc = include_str!("docs/tags.md")]
181pub use poem_openapi_derive::Tags;
182#[doc = include_str!("docs/union.md")]
183pub use poem_openapi_derive::Union;
184#[doc = include_str!("docs/webhook.md")]
185pub use poem_openapi_derive::Webhook;
186pub use validation::Validator;
187
188#[doc(hidden)]
189pub mod __private {
190 pub use indexmap;
191 pub use mime;
192 pub use poem;
193 pub use serde;
194 pub use serde_json;
195
196 pub use crate::{auth::CheckerReturn, base::UrlQuery, path_util::join_path};
197}