rs_opw_kinematics/lib.rs
1 2 3 4 5 6 7 8 9 10 11 12 13 14 15 16 17 18 19 20 21 22 23 24 25 26 27 28 29 30 31 32 33 34 35 36 37 38 39 40 41 42 43 44 45 46 47 48 49 50 51 52 53 54 55 56 57 58 59 60 61 62 63 64 65 66 67 68 69 70 71 72 73 74 75 76 77 78 79 80 81 82 83 84 85 86 87 88 89 90 91 92 93 94 95 96 97 98 99 100 101 102 103 104 105 106 107 108 109 110 111 112 113 114 115 116 117 118 119 120 121 122 123 124 125 126 127 128 129 130 131 132 133 134 135 136 137 138 139
//! Rust implementation of inverse and forward kinematic solutions for six-axis industrial robots
//! with a parallel base and spherical wrist
//!
//! This work builds upon the 2014 paper titled _An Analytical Solution of the Inverse Kinematics Problem of Industrial
//! Serial Manipulators with an Ortho-parallel Basis and a Spherical Wrist_, authored by Mathias Brandstötter, Arthur
//! Angerer, and Michael Hofbaur. The paper is available on [ResearchGate](https://www.researchgate.net/profile/Mathias-Brandstoetter/publication/264212870_An_Analytical_Solution_of_the_Inverse_Kinematics_Problem_of_Industrial_Serial_Manipulators_with_an_Ortho-parallel_Basis_and_a_Spherical_Wrist/links/53d2417e0cf2a7fbb2e98b09/An-Analytical-Solution-of-the-Inverse-Kinematics-Problem-of-Industrial-Serial-Manipulators-with-an-Ortho-parallel-Basis-and-a-Spherical-Wrist.pdf).
//! Additionally, it draws inspiration from a similar C++ project,
//! [Jmeyer1292/opw_kinematics](https://github.com/Jmeyer1292/opw_kinematics), which served as a reference
//! implementation for generating data for the test suite. This documentation also incorporates the robot diagram
//! from that project.
//!
//! # Features
//!
//! - All returned solutions are valid, normalized, and cross-checked with forward kinematics.
//! - Joint angles can be checked against constraints, ensuring only compliant solutions are returned.
//! - To generate a trajectory of the robot (sequence of poses), it is possible to use "previous joint positions" as
//! additional input.
//! - If the previous joint positions are provided, the solutions are sorted by proximity to them (closest first).
//! It is also possible to prioritize proximity to the center of constraints.
//! - For kinematic singularity at J5 = 0° or J5 = ±180° positions, this solver provides reasonable J4 and J6
//! values close to the previous positions of these joints (and not arbitrary ones that may result in a large jerk
//! of the real robot).
//! - Jacobian, torques, and velocities
//! - The robot can be equipped with the tool and placed on the base, planning for the desired location and orientation
//! of the tool center point (TCP) rather than any part of the robot.
//! - Experimental support for parameter extraction from URDF.
//!
//! # Parameters
//!
//! This library uses seven kinematic parameters (_a1, a2, b, c1, c2, c3_, and _c4_). This solver assumes that the arm is
//! at zero when all joints stick straight up in the air, as seen in the image below. It also assumes that all
//! rotations are positive about the base axis of the robot. No other setup is required.
//!
//! 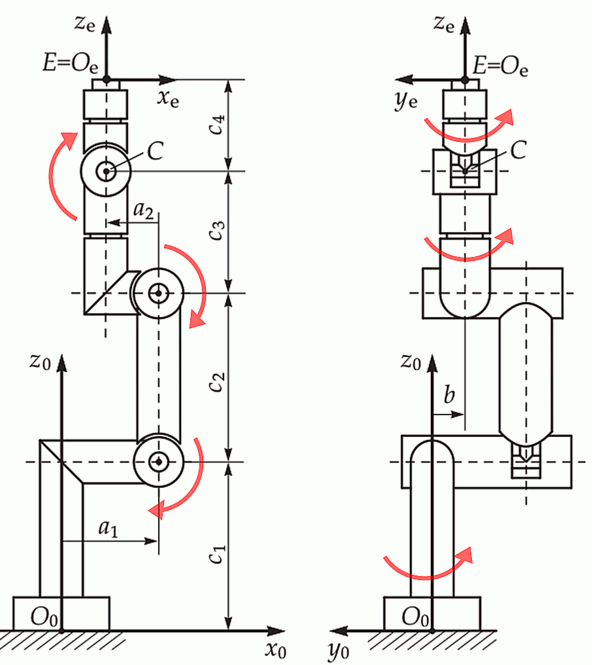
//!
//! To use the library, fill out an `opw_kinematics::Parameters` data structure.
//!
//! ## Examples
//!
//! The following examples demonstrate various functionalities provided by this crate:
//!
//! - **basic.rs**: Basic inverse and forward kinematics, including handling of singularities.
//! - **constraints.rs**: Limiting the rotation range of robot joints.
//! - **jacobian.rs**: Calculating Jacobian matrices for kinematic analysis.
//! - **paralellogram.rs**: Supporting robotic arms with a parallelogram mechanism.
//! - **tool_and_base.rs**: Configuring robots with a tool attachment and positioning on a specified base.
//! - **frame.rs**: Using frames, a foundational concept in robotic programming for managing coordinates.
//! - **complete_visible_robot.rs**: Constructing a complete robot with both shape and kinematics, including visualization.
pub mod parameters;
pub mod parameters_robots;
#[cfg(feature = "allow_filesystem")]
pub mod parameters_from_file;
#[path = "utils/utils.rs"]
pub mod utils;
pub mod kinematic_traits;
pub mod kinematics_impl;
pub mod constraints;
pub mod tool;
pub mod frame;
pub mod parallelogram;
pub mod jacobian;
#[cfg(feature = "allow_filesystem")]
pub mod urdf;
#[cfg(feature = "allow_filesystem")]
pub mod parameter_error;
#[cfg(feature = "allow_filesystem")]
#[path = "utils/simplify_joint_name.rs"]
mod simplify_joint_name;
#[cfg(feature = "collisions")]
pub mod collisions;
#[cfg(feature = "stroke_planning")]
#[path = "path_plan/cartesian.rs"]
pub mod cartesian;
#[cfg(feature = "collisions")]
pub mod kinematics_with_shape;
#[cfg(feature = "allow_filesystem")]
#[path = "utils/read_trimesh.rs"]
pub mod read_trimesh;
#[path = "visualize/visualization.rs"]
#[cfg(feature = "visualization")]
pub mod visualization;
#[path = "visualize/camera_controller.rs"]
#[cfg(feature = "visualization")]
mod camera_controller;
#[cfg(feature = "stroke_planning")]
#[path = "path_plan/rrt.rs"]
pub mod rrt;
#[cfg(feature = "stroke_planning")]
#[path = "path_plan/rrt_to.rs"]
mod rrt_to;
#[cfg(feature = "stroke_planning")]
#[path = "path_plan/altered_pose.rs"]
mod altered_pose;
#[cfg(test)]
#[cfg(feature = "allow_filesystem")]
mod tests;